In this tutorial I’ll be showing you how to add category archive pages to our AngularJS WordPress theme. Topics will be covered including:
- Get categories with WP REST API
- Display category links
- Create the route to category pages
- List posts in a certain category
- Replace the current category link with plain text to improve the usability
- Bonus: Display the featured image in posts list
- Bonus: Use JSON Formatter to improve the readability of JSON data and help you debug
- Updated 04/11: Get category archive page with slug
Before we get started, I hope you’ve read some tutorials from my Building themes with AngularJS and JSON REST API series, so we can be on the same page later when we dive into the code. If you’re new here, I’ll recommend that you start from the first post in this series: Using AngularJS and JSON API in Your WordPress Theme, which is listed on the Resources page at wp-api.org. It will definitely help you to build a quick and dirty AngularJS WordPress theme, so please go check it out.
When I was talking about adding category archive pages to our project, I meant you’ll make the theme work like this:
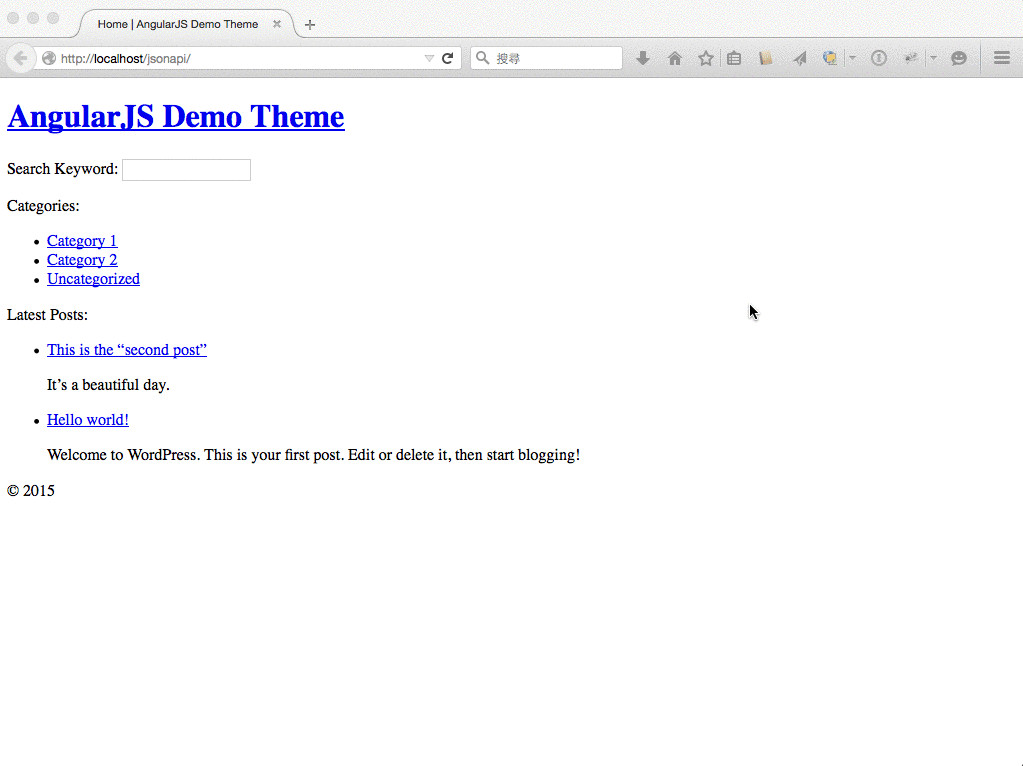
I know it didn’t look too impressive but now you get the idea. You can grab a copy of my AngularJS demo theme and let’s write the first line of code to get categories with WP REST API.
1. Getting categories with WP REST API
To get all categories from your WordPress site with WP REST API, we’ll modify our Main controller to the following code:
In line 7-9, you can see the endpoint looked like this:
wp-json/taxonomies/category/terms
With such format we can also get all tags with the following endpoint:
wp-json/taxonomies/post_tag/terms
And you might notice that in line 13 I add a new property to $scope
object called “pageTitle
“, which I’ll use to make the pages more distinguishable later when we switch from homepage to a category page.
2. Display category links
Since we’ve added the categories
property to the $scope
object, now we can use it in our main.html
.
We can loop the categories just as we looped the posts – with the lovely ng-repeat
. In line 5 I set the url href to the category page in such format:
category/{{category.ID}}
So in next step, we’ll need to create a corresponding route to make it work. And just a quick note, I’ve added the {{pageTitle}}
in line 8 to display a simple page title here.
3. Create the route to category pages
At this point, you should be familiar with AngularJS route that every time when we create a new view (a new page), there must be a corresponding route. By doing so, AngularJS can get the right view for us when the route (URL) changes.
In line 16-19 is the new route I created for category pages. Please note the routeParam :category
is the category ID.
In this route I used the same template file as the homepage, but created a new controller called Category
. In the Category
controller we’ll get posts in the category, and we’ll add a new property to the $scope
object to identify the current category.
4. List posts in a certain category
You can see in the Category
controller, I kept the same snippet to get all categories in the Main
controller, so I can still list all categories in a category page.
In line 8, I used the endpoint in WP REST API to get a certain category object by it’s ID:
'wp-json/taxonomies/category/terms/' + $routeParams.category
The only reason I did this is to get the name of the category, which is res.name
that I put in pageTitle
and document title. I even used it to get posts belong to it.
In line 13, you can see the endpoint in WP REST API to get posts from a certain category is wp-json/posts/?filter[category_name]=category_name
.
Now I’ve passed everything the view (main.html
) needs, it’s time to improve the view.
P.S. In line 9 I added a new property to $scope
called current_category_id
, which would be very useful in next step.
5. Replace the current category link with plain text to improve the usability
Now you can test to see if the category route and controller work. When clicking “Category 1” link, you should see the Category 1 archive page like this:
In such view the best practice regards to the usability, is that we should remove the link of the current category, so our users can be less confused (even though a experienced user would tell from the color of the links).
To replace the current category link with plain text (only category name), I’ll introduce a new built-in directive in AngularJS called ng-if
.
It’s obvious that ng-if
can be used to help us hide or show something in certain conditions. Check the line 6-7 to see how I set the conditions that show or hide category links. Hint: the current_category_id
played a very important role.
6. Bonus: Display the featured image in posts list
Since we talked about ng-if
, another example to use it is to display the featured image.
With ng-if
the featured image only shows when it’s available, like the following screenshot:
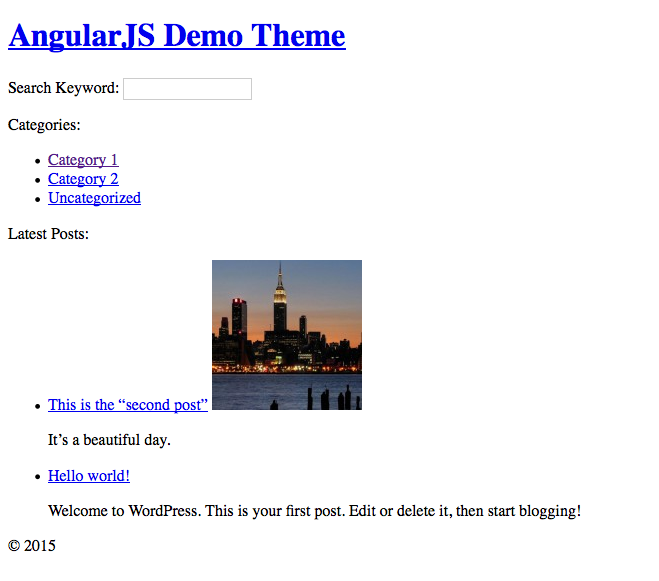
In line 5 I’ve also introduced another new built-in directive called ng-src
, which is very helpful when the image path contains AngularJS markup like {{path}}. Because browsers will fetch from the URL with the literal text {{path}} (rather than the rendered value) if we put the {{path}} to src
attribute.
7. Bonus: Use JSON Formatter to improve the readability of JSON data and help you debug
Whenever I needed to test if an endpoint in WP REST API works, I always entered the URL in browser and then viewed the JSON data.
After a while I felt really frustrated that the JSON data always looked messy and didn’t really help debugging. If you have the same feelings, I believe the JSON Formatter extension (it’s for Chrome, but you must can find similar extensions for Firefox) is all what we need.
The JSON data from the WP REST API will look gorgeous after we install the JSON Formatter:
8. Updated 04/11: Get category archive page with slug
Starting from WP REST API 1.2, we can get terms with slug by passing the filter
argument. For example, we can call the API with /wp-json/taxonomies/category/terms/?filter[slug]=uncategorized
to get the uncategorized category, which would be an array contains the term object.
For this matter now we can get category archive page with slug. Let’s update our scripts to do so.
First, we’ll change the endpoint to get the category (at line 9). And because now we get the result as an array, we need to update all res
object to res[0]
.
Then we update the link href in main.html
, we change the link from “category/{{category.ID}}” to “category/{{category.slug}}
“. And everything will work just as we expected.
Last but not least is to change the “Category base” to “category” at the Permalink Settings page. By doing so we can make the default permalink to category archives in WordPress to be “[site-url]/category/%category%/”, rather than “[site-url]/blog/category/%category%/”, and it will consist with the category route path in our AngularJS app.
Whenever we add new routes in our AngularJS app, we’d better to make sure that the route paths consist with the permalink structures in WordPress. Because search engines are good at following the default URL structure generated by WordPress, and if they visit a URL doesn’t match any route paths in our AngularJS app, they will be redirect to the default route path (homepage in our case). If there are too many pages are redirected to homepage, you’ll get into trouble on SEO performance.
Now you can get the whole project files from the Github repo. I hope you enjoy this tutorial and learn something valuable. If you still get confused by some AngularJS terms or concept, be sure to check out the post Minification Errors in My AngularJS WordPress Theme, that I kind of summed up the first 3 tutorials in my AngularJS series to help readers to get a clear picture.
If you bump into any issue related to this tutorial, please don’t hesitate to contact me. I’d love to get back to you as soon as I can.
Leave a Reply